The C++ programming language is one of the most important and common tools in a modern developer's repertoire. Although it is less popular than Java or Python (according to the Stack Overflow Developer Survey, discussed below), C++ code is found in nearly every current operating system (OS), web browser, game and office productivity suite.
C++ is an extension of the C programming language and uses syntax similar to C, C# and Java, making it reasonably straightforward to learn for new developers and experienced programmers alike. Like the other languages, the syntax can be complex.
This article explains what C++ is and how to start learning it. It also compares C++ to other common programming languages so you can decide whether it's a skill you need to add to your knowledge base.
Here are a few common attributes of C++:
- Generic, imperative and object-oriented programming language
- Used in developing cross-platform applications due to its portability and platform adaptability
- Used to create high-performance applications
- An extension of the C programming language that supports objects and classes (C does not)
- Offers developers lots of control over how the application consumes resources
Many of today's most prominent applications—along with all three major operating systems—use C++ code to some degree (often mixed with C code). Examples include Microsoft Office, World of Warcraft, Photoshop and even the Chrome, Safari and Firefox browsers. Windows, macOS and Linux contain significant amounts of C++ code as well.
C++ Key Features
Let me be clear: C++ is an advanced programming language with a steeper learning curve than a language like Python. That's not a bad thing, as it gives developers control over the system running the application to create complex and powerful programs – just be aware of the commitment involved.
Developers use object-oriented languages to define classes. Classes are items containing one or more attributes. Instances of the classes are known as objects, which can be manipulated in the code. Many languages use this approach, including C++.
Like other languages, C++ uses libraries to make coding more efficient and consistent. Libraries are collections of code written to achieve common or standard programming needs. Consider how often a developer might want the code to perform mathematical functions or collect and manipulate data. These are frequent requirements, so rather than each developer worldwide having to recreate this code over and over, libraries exist that programmers can call in their code to accomplish these standard tasks.
Here are a few C++ libraries with descriptions:
- General utilities: Supporting elements for other libraries
- Memory management: Components to help set memory controls
- Diagnostics: Error-reporting library
- Numerics: Complex mathematical functions
Various training opportunities exist online or in-person to help you start with C++. It may be worthwhile to explore those, especially if you're new to programming. Below are the tools you need to get started and some projects to consider.
C++ Integrated Development Environments and Text Editors
One important set of tools for learning and using any programming language is integrated development environments, or IDEs. These dedicated programs include editing, debugging, compiling and syntax-checking features. Capabilities vary widely, but many IDEs support multiple languages and are extensible. They also have various learning curves. Finally, some are free, and others have a subscription or fee.
Eclipse
One popular and free IDE is Eclipse. It supports several programming languages, including Java, JavaScript, PHP and C/C++. You can rely on strong community support and many plugins. It may be resource-intensive on your system.
Code::Blocks
The Code::Blocks IDE is free. It's designed specifically for C, C++ and Fortran projects. Its array of plugins makes it customizable and extensible. Support includes online forums, a wiki and a user manual. The following image shows a demonstration file and the default interface:

Visual Studio/Visual Studio Code
Microsoft's Visual Studio is a powerful IDE on developer desktops. It focuses on .NET and C++ development. One strong feature is its graphical design program—perfect for those who need to visualize coding projects. Visual Studio comes in three editions: Community, Professional and Enterprise. Technically, Visual Studio Code is a text editor. I mention it here because it's closely associated with the Visual Studio IDE.
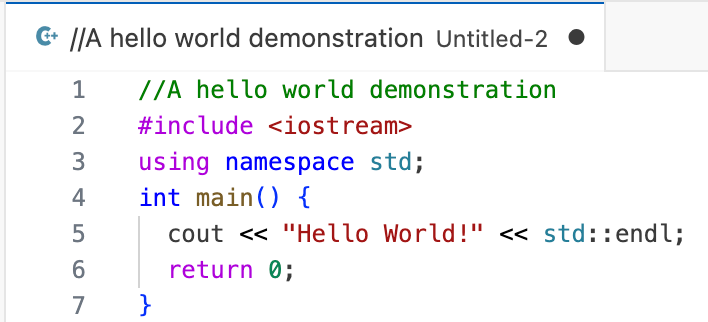
Text Editors
However, if you'd rather start simple, any basic text editor will do for writing code. I suggest the open-source Vim editor. Windows users can consider Notepad++ as an option. Don't use a word processing program like Microsoft Word or Libre Writer. These powerful tools have a different purpose, and they insert formatting and other instructions behind-the-scenes that do not play well with code.
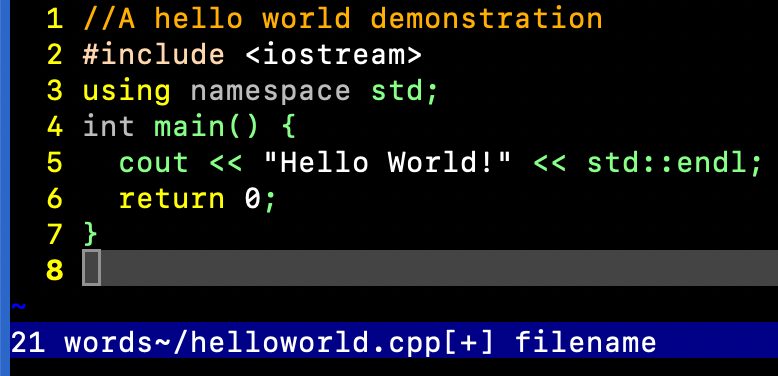
When to Use C++
C++ pervades major application development projects. These include full operating systems, performance-oriented applications or web browsers. You'll probably need to use C++ in organizations that create applications in-house or write extensive code.
C++ is not really a hobbyist language; most people who master it do so to expand their career opportunities. In other words, your goal for learning C++ is likely to either find a job with a major application developer or enhance your skills for your existing programming role.
Some C++ Project Examples
Many people exploring the IT field want to experiment before committing to expensive and time-consuming training (whether self-taught or through a training provider). In particular, developers tend to be self-motivated, inquisitive and problem-solvers. Therefore, consider a few of the projects below to see whether C++ intrigues you:
- Basic game: Find a tutorial for a basic game, such as rock-paper-scissors.
- Inventory management: Create an inventory management system for your book collection (or some other collection).
- Authentication application: Build an application that checks credentials before allowing access to a resource. Remember, this simulates a real authentication system—you don't need anything extensive for it to control access to.
- Text editor: Find instructions for creating your own text editor and extend its functionality over time. You could even release it as your own piece of open-source software!
- Web browser: For the adventurous (and more advanced), build your own web browser.
Many tutorials and guides exist online, and you don't need much to begin working with C++ code.
Get Started With C++
Ready to experiment with C++? Technically, you only need a text editor and a compiler to begin. However, using a dedicated IDE with a built-in compiler, command suggestions and syntax-checking features may be better. Download one of the free tools I linked above.
Note: Various online IDEs allow you to edit and run code without installing anything on your local system.
I'll use the standard "Hello World!" demonstration to show a few basic C++ techniques. Open a text editor, name it helloworld.cpp, and add this code:
//A hello world demonstration
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << std::endl;
return 0;
}
C++ ignores spaces in the code, such as those used to indent some of the lines above. They exist to make the code more readable. Notice the semi-colons at the end of each statement and the curly brackets enclosing the function. These are vital delimiters as the code grows longer and more complex.
Comments allow developers to put instructions or other information in the code without the system attempting to run the content. Comments are an easy way to add examples or display options. They are also useful in troubleshooting as a way to prevent certain blocks of code from running. Comments in C++ are marked with //. Multiple lines of comments are bracketed by /* and */. Note that this is the same comment syntax used by JavaScript, among others.
Here are the steps to start compiling the code you wrote above. The examples are from a Linux system using a text editor (Nano) and the GCC compiler.
The -o helloworld string below defines the name of the compiled program. The helloworld.cpp file name represents the code you wrote that you want to compile. You can run the program using the Linux ./helloworld command. Recall that an IDE handles this for you, simplifying the process.
1. Install GCC using the DNF package manager:

The installation may take up to two minutes. Depending on your environment (operating system, IDE, online IDE, etc.), you may already have a compiler installed. If so, compiling a C++ application at the command line is straightforward.
2. Compile the C++ code. Specify the file with the .cpp file extension and provide a name for the new executable application by using the -o option:
$ g++ helloworld.cpp -o helloworld

3. On a Linux system, use the chmod command to make the program executable:
$ chmod 755 helloword

4. Run the helloworld application. Be sure to use ./ in front of the application name so Linux checks the current directory:
$ ./helloworld

The GCC compiler even has basic debugging features. In the following example, I removed the mandatory ; from the end of line five:
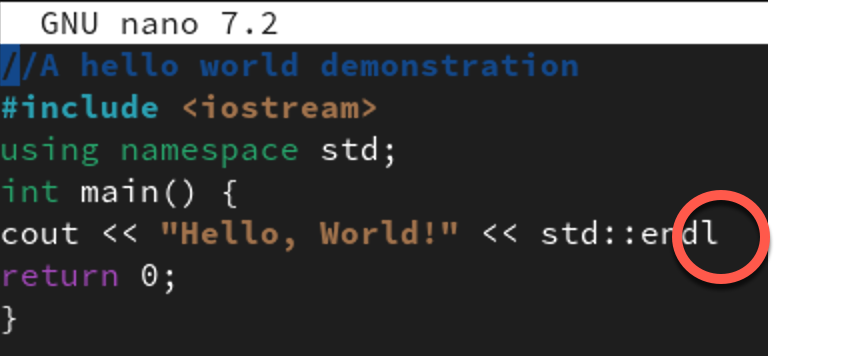
I tried to compile the program and received the following message:
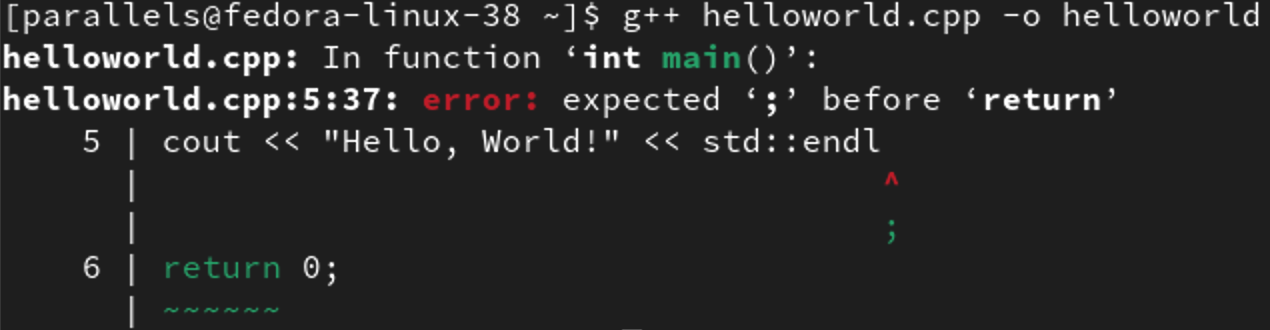
A handy feature!
C++ Versus Other Programming Languages
C++ compares well with other languages, but like any coding choice, the best solution depends on the problem being solved. C++ isn't for every development project.
So why choose C++ as your next (or first) programming language? It's robust enough to give you a solid understanding of other languages you might encounter later. You'll learn all the fundamentals—from variables to loops to functions—to deliver solid, functional code. C++ gives you experience with resource utilization in application development, especially memory management.
The 2023 Stack Overflow Developer Survey ranks C++ as the ninth most popular language. It doesn't score as well as Python, JavaScript or Java, but it's important to remember the size of the projects where C++ is most commonly found. It targets a different workload than some of the other languages or tools. And number nine out of ten is not a bad position.
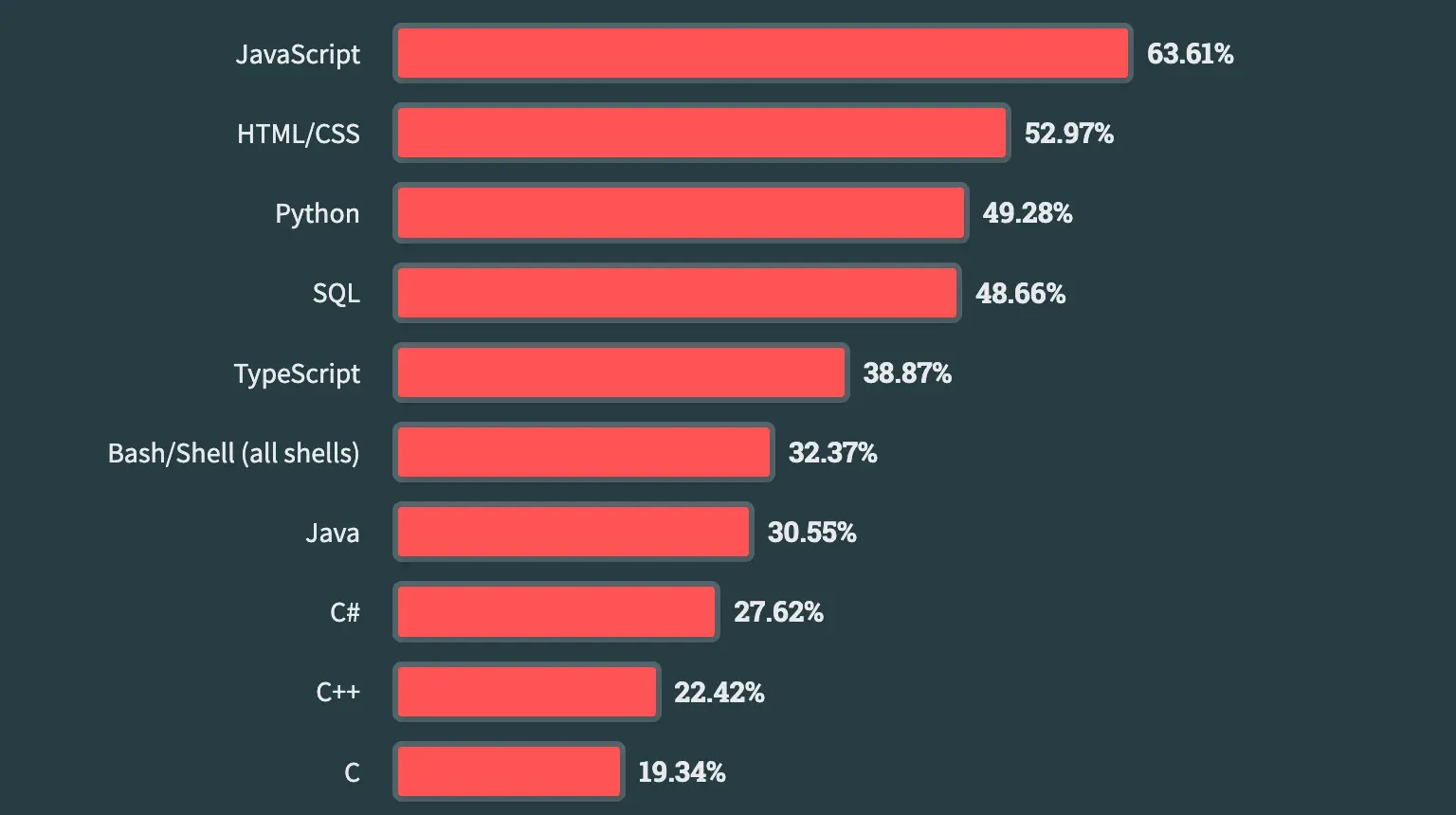
C++ Advantages and Disadvantages
C++ is fast, powerful and flexible, but those traits lead to a significant learning curve. Here are some advantages and disadvantages of C++.
The Advantages:
- C++ is multi-paradigm supporting generic, imperative and object-oriented design paradigms
- Efficient for general applications
- Provides control over resource management, providing efficient code
- A robust and large community offers a broad selection of libraries
- Portable across various hardware and OS platforms
The Disadvantages:
- Complex and difficult syntax
- Contains security issues
- Some issues are difficult to debug
C++ is a vital language in today's development environment. Operating systems and applications written with C++ exist on nearly every device. The syntax is related to that of C, C# and Java, but that syntax is also complex. Still, if you're pursuing a career in programming, C++ provides the concepts and skills you need to be successful and understand other languages.
Get started today by choosing a C++ IDE, finding a simple tutorial or project and building your knowledge.
Learn the skills you need with CompTIA CertMaster Learn. Sign up today for a free trial today!