JavaScript is an essential scripting language for today's web development. Nearly every website you visit uses JavaScript in some way. It's JavaScript that makes sites interactive and dynamic, something today's web users have come to expect.
Web pages are constructed using three basic tools:
- Hypertext Markup Language (HTML): Language to format web pages, including headers, lists, image locations, text blocks, etc.
- Cascading Style Sheets (CSS): Language describing how HTML information will be presented on screen to users
- JavaScript: Scripting language to provide dynamic content in web pages
JavaScript provides the dynamic and interactive content most of us expect from modern websites. Want to push a button to access information? That's JavaScript. Need to convert two different currencies, or maybe convert between Celsius and Fahrenheit? That's JavaScript.
This article provides basic concepts and ideas to get you started with JavaScript. If you intend to learn JavaScript, then be sure to read up on HTML and CSS, too. The three are closely linked.
Key Features of JavaScript
I'll identify some key features of JavaScript before getting into the details of syntax and projects.
First, I'll clear up a common misunderstanding that occurs between JavaScript and Java. These are not the same languages and only bear a passing resemblance to each other. Java is an object-oriented programming language used to build applications that run on almost any platform. Its use and structure are significantly different from JavaScript. JavaScript is an interpreted language (meaning it is not precompiled), and its code is relatively easy to read and understand. Java code runs inside a Java Virtual Machine (JVM), while JavaScript is usually executed via your web browser.
JavaScript almost always executes on the client system rather than on a remote web server. When you access a website that contains JavaScript code, your system downloads the script and runs it locally, using your computer's processing power. The same occurs with the HTML and CSS code that makes up the other parts of the website.
If you're reading this, you are probably interested in learning a programming language. You may wonder which to choose—there are so many options! JavaScript is a common choice, as is Python. The real decision comes down to function: Why do you want to learn a language? The reason is usually to solve a problem or possess a skill for career development. JavaScript is mainly found in web development, and Python is generally more of a jack-of-all-trades. If web development interests you, JavaScript is the way to go.
The formal name for JavaScript is ECMAScript, and its current version is ES14. Each iteration includes functional improvements.
JavaScript Integrated Development Environments
Developers may use almost any text editor to write JavaScript code. In fact, many developers rely extensively on Vim, Notepad++ and Sublime. These tools are highly extensible and customizable, but they are mainly just used to generate basic text.
More feature-rich integrated development environments (IDEs) provide additional functionality, including:
- Syntax checking specific to one or more languages
- Build tools
- Debugging capabilities
- Source and version control features
If you'd like to use an IDE to begin your JavaScript journey, here are a few good options:
AWS Cloud9
One solution for JavaScript development is Amazon's AWS Cloud9. This cloud-based IDE does not require you to install any applications locally; everything happens in the cloud. One great result is integrated collaboration, making both development and debugging easier. AWS Cloud9 is intuitive and easy. It's available from anywhere (if you have a good internet connection). Be aware that AWS Cloud9 isn't free and requires an AWS account.
Webstorm
A leading JavaScript editor is Webstorm from JetBrains. Webstorm is available for Linux, macOS and Windows, and JetBrains offers a 30-day free trial. Webstorm was developed specifically for JavaScript and related technologies. It includes syntax checking, autocompletion, suggestions and collaboration options. A Webstorm subscription isn't cheap ($159 for one user for the first year), but it is certainly feature-rich.
Many other full-featured examples exist, but these are plenty to get you started. Don't forget that you can do all you need with tools like Vim and Notepad++.
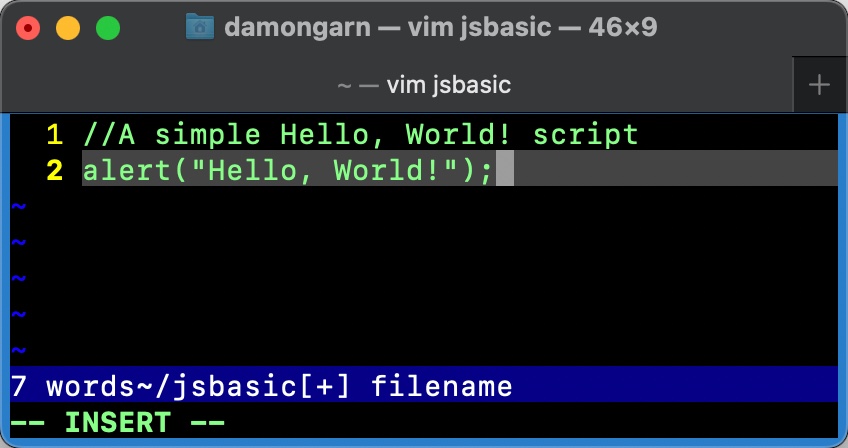
JavaScript Project Examples
If you're interested in working with JavaScript, one good approach is to find small, basic tasks or projects to work with. Begin with very simple ideas and progress toward the more complex. Learning a language is difficult and takes time. Programming tutorials often use games as examples.
Here are a few project examples you could look for:
- Number guessing game
- Number incrementing game
- Daily task list
- Countdown timer
Common Concepts
Most programming languages must solve similar problems. They may need to store information for use later, solve basic math equations, accept user input or request specific resources. Because of this, many concepts are similar among languages.
What Is a Statement?
Applications consist of one or more sets of instructions for the computer to run. These instructions—called statements—are built in different ways, depending on the language used.
JavaScript delimits statements using the semi-colon character (;), like this:
alert("Hello, World!");
Think of these statements as the basic structure of the JavaScript code. You'll use specific components, such as values, operators and keywords, to construct the functionality your program requires.
Code Block
Some statements are very short; others are much longer. Several statements may be grouped together into code blocks. The blocks are enclosed in curly brackets ({}). Functions are an example of code blocks.
Spaces
Some instruction sets, such as YAML (Yet Another Markup Language), use spaces to define statements or other sections. JavaScript does not recognize spaces or white space as delimiters. Instead, developers combine comments with white space to make the code more readable.
Notice there are no line breaks in the following example, making it more difficult to read:
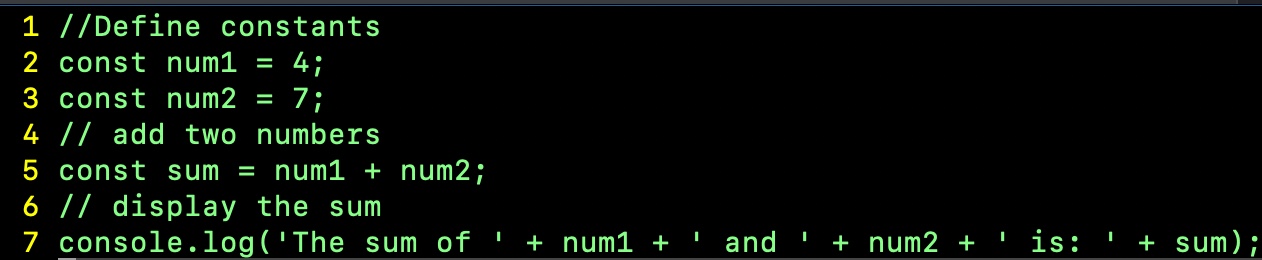
The following code includes line breaks between some line numbers, making the code sections easier to identify:
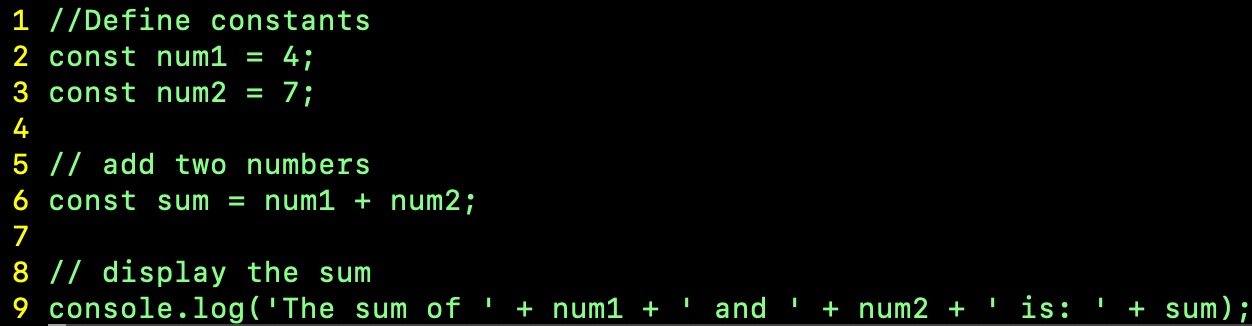
Comments
Like other scripting and programming languages, JavaScript supports comments. Comments allow developers to insert instructions, examples, explanations and other human-useful information into the body of the code. Comments are an important way of explaining to others (or reminding yourself) why something is the way it is in the code. While many languages use the hash character (#) for comments, JavaScript uses two forward slashes (//).
// comment example
This screenshot appeared above, but I want to call out the comment on line 1 specifically:
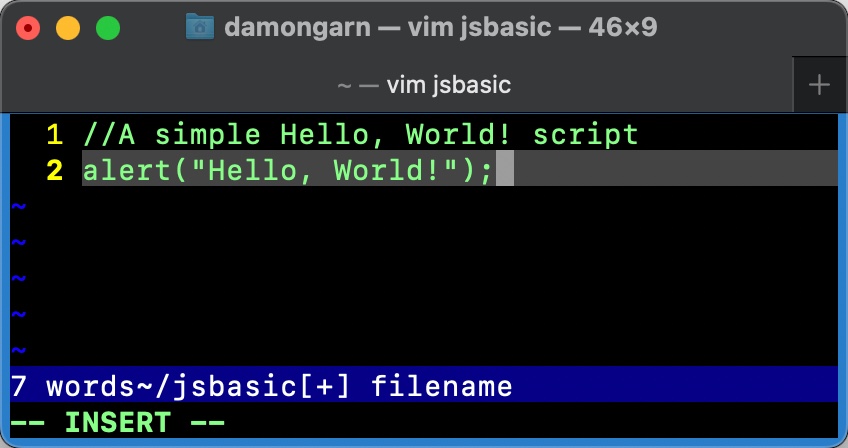
Notice the comment explains the function of the alert(); code following it. If a comment will span more than one line, the format is slightly different:
/*
multiline example
line 1
line 2
line 3
*/
In the following image, the comments span two lines:
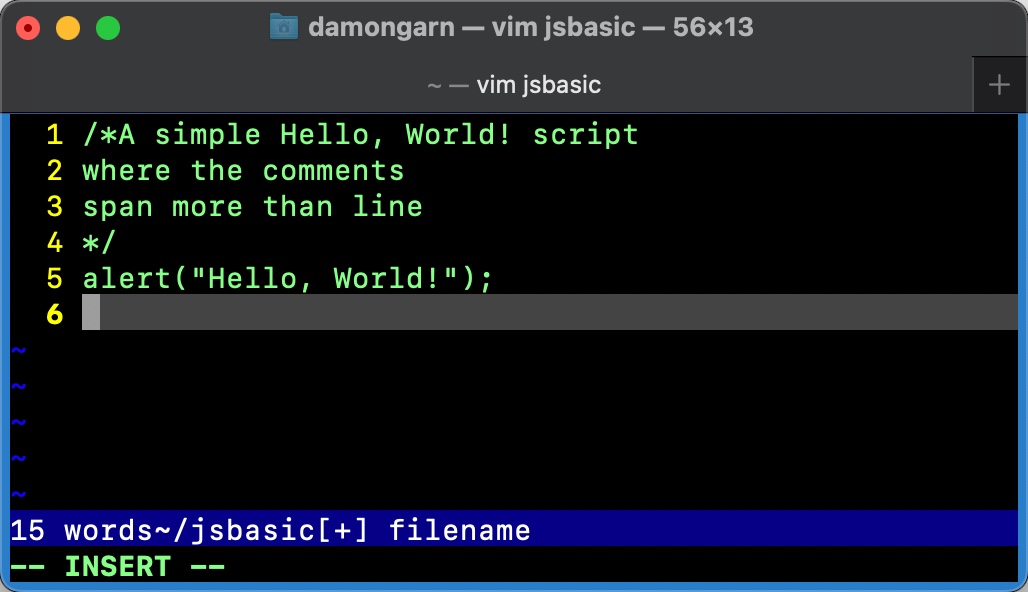
It's a good idea to get in the habit of using comments in any coding project. It's even a useful practice with configuration files.
Value
Values are entities stored within literals or variables. These values may be text, numbers or other data.
Literals
JavaScript uses literals to recognize information that is static or does not change. These are set with no leading information. The first example below shows a fixed numeric value, and the second shows a fixed text value:
42
"Hello, World!"
Variables
JavaScript stores information in variables for use later in processing. Variables might include values such as numbers, names, product ID codes or other information.
JavaScript recognizes four ways of assigning variables:
- ·var
- let
- const
- Automatically (when first used)
Here's an example of basic math using the var keyword:
var x = 22
var y = 20
var z = x + y
Variables may store strings (text information), such as names or other words. These data types are enclosed in double quote characters (").
Operators
Like other languages, JavaScript can perform math functions on data, including adding, subtracting, multiplying and dividing. It also does more complex operations, such as comparisons or logical operations.
Operator | Operation |
+ | Perform addition |
- | Perform subtraction |
* | Perform multiplication |
/ | Perform division |
++ | Increment a value |
-- | Decrement a value |
Key Words
JavaScript reserves the use of 63 specific words for particular capabilities. Examples include var, const, for, while and many others.
Saving Scripts
JavaScript statements may become too large to embed directly in an HTML document. Instead, save the code into a text file containing the .js extension and call the file from within the code. Here is an example:
<script src=/path/to/script.js></script>
Get Started With JavaScript
It's time to get started! JavaScript is a great way to learn some basic programming concepts. You won't need to install much—your system probably has a basic text editor and web browser already installed. You'll use the text editor to write code and the web browser to observe the results.
Common text editors:
- macOS: TextEdit
- Linux: Gedit, Vim or Nano
- Windows: Notepad (though I suggest you download Notepad++)
Note: Don't use a word processing program like Microsoft Word or LibreOffice Writer. These programs are very sophisticated and add additional formatting and other instructions to the document that interferes with coding.
Here is an example of using Vim on my Mac for JavaScript and HTML. Write the following into a text document, save it as a script and call the script through a web page.
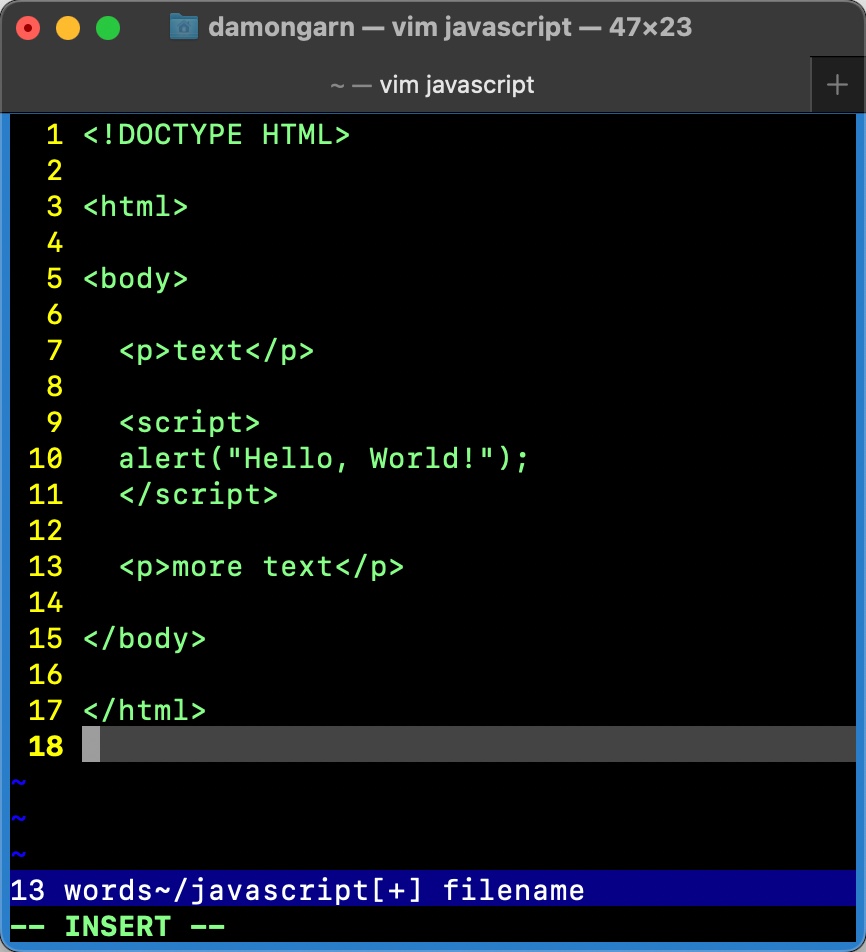
JavaScript Versus Other Programming Languages
The 2023 Stack Overflow Developer Survey ranks JavaScript as the most popular language. Notice that HTML/CSS are in second place. These three normally go together anyway. Python is ranked third and continues to grow in popularity.
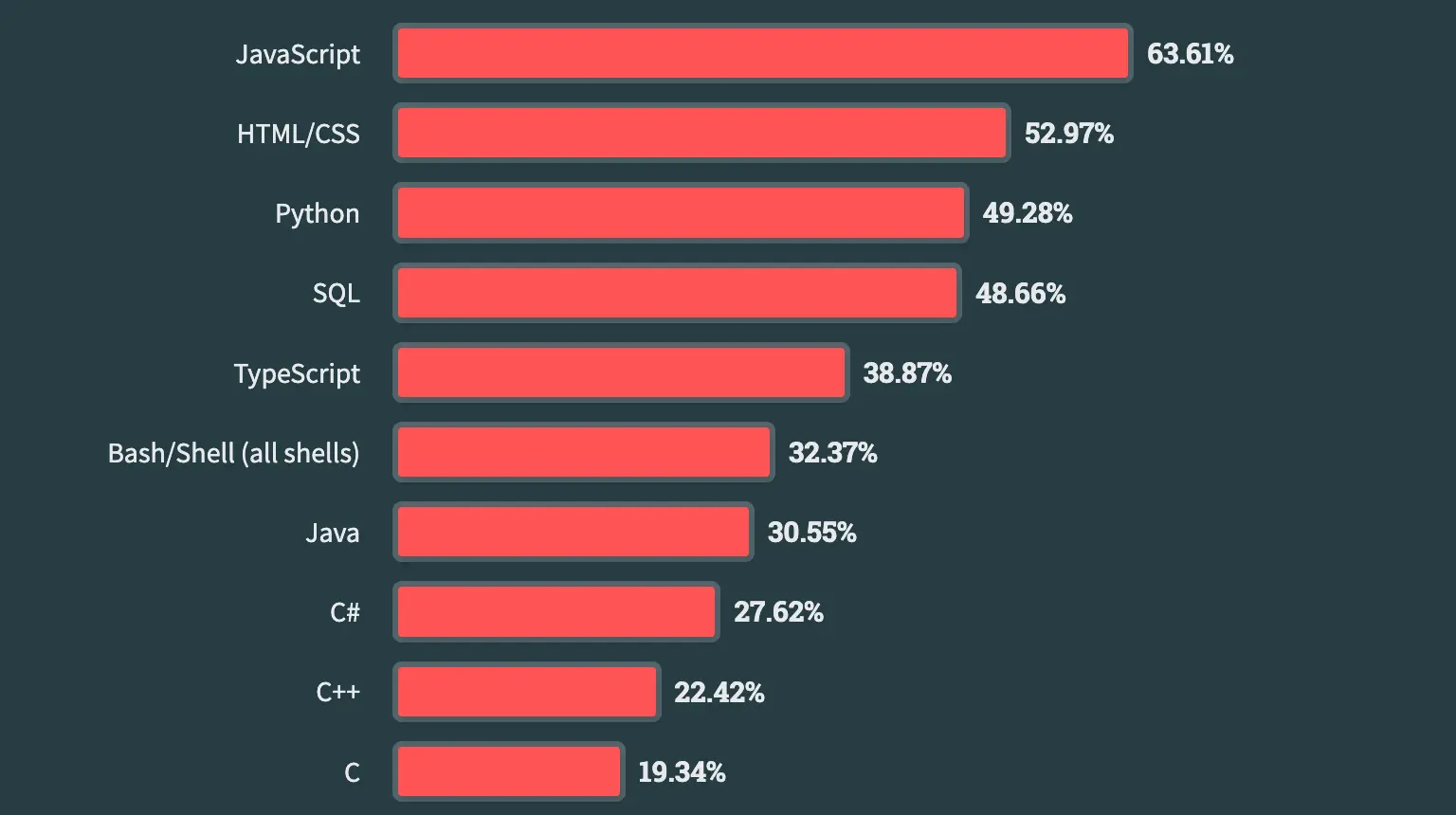
Many folks just learning a programming language wonder where to start. One common choice is Python, a powerful interpreted programming language found in many enterprise environments, especially science and machine learning. JavaScript is also a great place to start. Like Python, it is relatively simple to learn. Both have the advantage of being very common, meaning you will likely find a use (or employment) for both. They are not used to solve the same problems, however. JavaScript is mainly found in web development.
JavaScript also differs from more complex compiled languages, such as Java and C++. Not only is the syntax different, but those languages may be tougher to learn and have a different purpose (mainly the development of larger applications). JavaScript is primarily found in web design industries.
I suggest you choose a language based on your professional goals rather than popularity. More specifically, if you're doing website development, you'll almost certainly need JavaScript skills (along with HTML and CSS).
JavaScript Advantages and Disadvantages
Consider the following advantages and disadvantages of JavaScript.
Advantages:
- Large support community
- Large number of online resources
- Will continue to be in high demand for the foreseeable future
- Easy to learn and code
- Code readability for interpretation and debugging
- Cross-platform (Linux, macOS, Windows)
Disadvantages:
- Specific to web development (with a few exceptions)
- Probably requires a working knowledge of HTML and CSS
- Not as powerful as a compiled application
JavaScript for Website Development
It's essential to remember that JavaScript is a key tool for one job – websites. While JavaScript statements can run without being deployed to a site, dynamic web page presentation is really what JavaScript is all about. And JavaScript script execution typically occurs on the client system. If you want to learn this language, you're probably doing so for a specific professional need tied to website development. In addition, you should also familiarize yourself with HTML and CSS.
You now have a basic understanding of JavaScript, and your computer probably has all it needs. It's time to search for a fun and easy first project and get started.
Ready to upgrade your IT skills? We've got great news! You can save big on CompTIA certifications and training right now.
0